Lists in Python
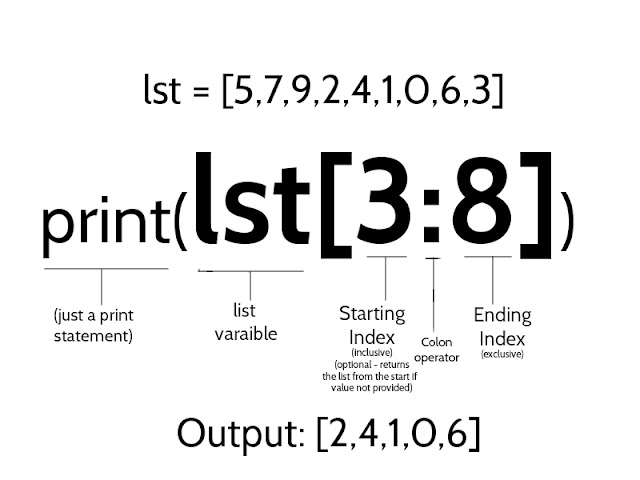
List in Python is a collection of similar/different data types. Defining a list: A List can be defined/created by enclosing the values within square brackets '[]'. l = [5,7,9,2,4] The above code will create a list with the values given inside the brackets. We can create an empty list by giving just nothing within those square brackets. l = [] Printing a list: We can print the list just by passing the list into the print() function. l = [5,7,9,2,4] print(l) Output: [5,7,9,2,4] Creating a list with predefined values: We can create a list with predefined values by using * operator on any list multiplied by n times. l = [0] * 5 print(l) Output: [0,0,0,0,0] Accessing list elements: We can access the elements of a list by calling the list followed by the index value enclosed in square brackets. l = [5,7,9,2,4] print(l[2]) Output: 9 Since Indices of a list start with 0, Index 0 corresponds to the first element in the list, Index 1